Let's Learn React the easy way but let's first try to understand what is react in very simple terms. It is not a framework, it is simply a javascript library. It is used to create interactive website interfaces which also allows us to easily create Single Page Apps - SPAs for short.
Contents
SPA (Single Page Application)
By single page application, we mean the server only ever needs to send a single html page to the browser for the website to run fully.
Traditionally, without react, every link a user clicks would send a request to the server and the server would look at the URL and then it would send back that html page, this would be the same for every single page.
React, what it does is, it takes over the whole website in the browser and manages the whole website in the browser including any kind of website data or user interactivity such as click events and even routing from page to page. For the navigation between different pages, we are not dependent on the server, React manages all of this on it’s own.
Getting started with React Application
There are few different ways to get started with react, but it is easier when we use a tool called create-react-app. It is a command-line tool which generates starter react project and it comes with all the setup that we need i.e babel and webpack. We need all of these things to convert our jsx code i.e react code into production ready javascript code. Without this tool, we would have to configure all of those things ourselves.
To run this tool on your computer, you need a modern version of node installed on your system. You can check that by going to command prompt or terminal and do as mentioned below and this will show your node version. Ideally, your node version should be 5.2 or above:
If you do not have the desired version or if you don’t have node installed you can go to nodejs.org and download the latest version.
Once you have installed node with the current version, you can navigate to the desired directory and create your project as mentioned below:
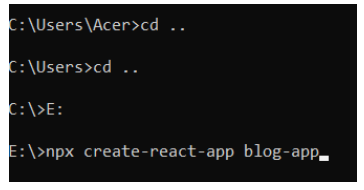
npx will pull out the latest version of create-react-app tool from internet and put all of those files in your application named blog-app.
CRA Architecture
The file structure looks like this:
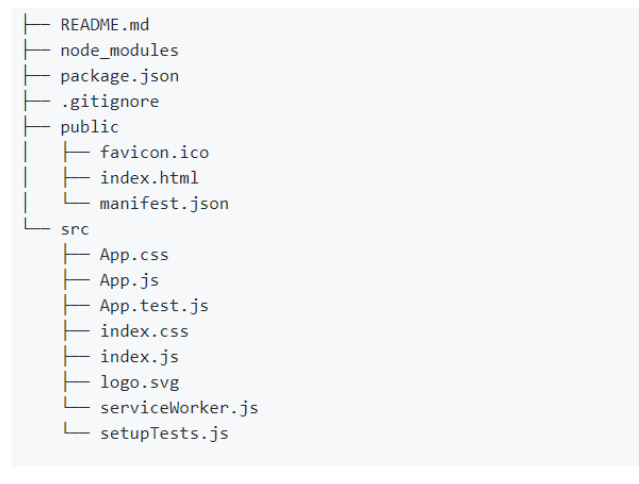
Let’s try to understand the CRA architecture in brief. We will understand the reason behind all of these things when we will learn about pure react in the upcoming topics. Let’s start from the node modules.
All the project dependencies lives inside node modules and any of the package that we install from the internet is also going to live inside it.
Public folder has got all the public files which are public to the browser including index.html file.
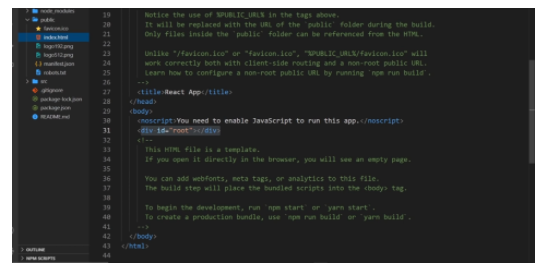
All of our react files is then injected inside div with id root .You can put some text inside it if you want to check whether your react code is being rendered into it or not. If the code is being rendered then any text you put inside that div would be replaced by that code.
Next is your source folder, almost 99 percent of your code that you write is gonna go inside your source folder. All of our components that we are going to write is going to live inside our source folder.
We have index.js file inside our source folder which going to kickstart our application. It is going to take all of our react components and mount it to the DOM.
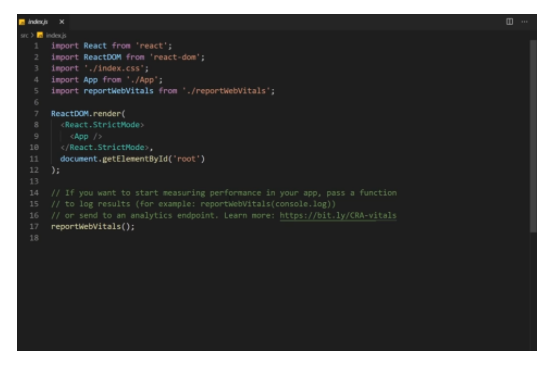
If you see clearly, it is taking our app component and rendering it to the DOM and it is rendering our app component inside the div with id root as discussed earlier. We have logo.svg which is being used inside our app component. We also reportWebVitals which is a performance file.
The files App.css, App.test.js, logo.svg, setupTests.js and reportWebVitals.js may be deleted as they are not needed in our application right now.
React.Strictmode means React does additional checks during development and it gives the warning down in the console if there are any warnings to report.
We also have gitignore file just in case we are using version control with git.
Now, coming to package.json, it lists down all the dependencies of the application . So, anything that our projects depends on which all lives inside node modules.
We also have scripts which can help us preview our application in a browser, on a local server or build our application or build our application for production or test our application or eject the webpack file.
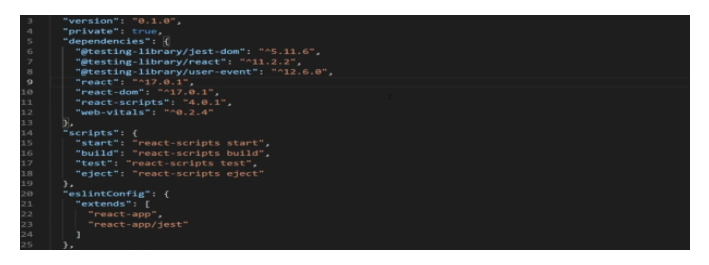
The application runs as follows:
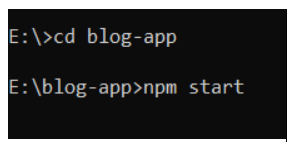
By default, the application runs in localhost port 3000 with the address http://localhost:3000 and looks like as follows:
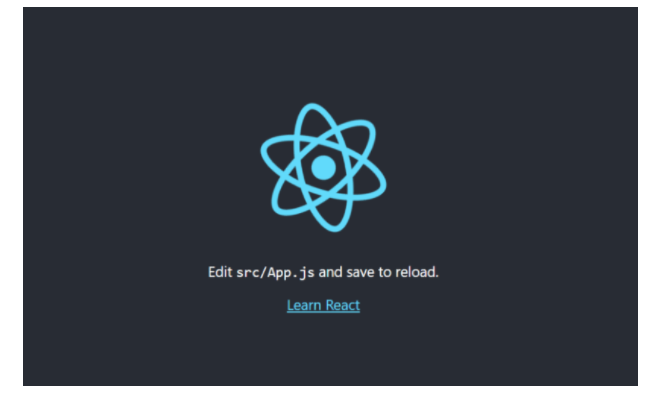
This is a live reload server. It means if you make some changes and save it in the editor .It will hot refresh in the browser.
Let’s say you are starting out with some projects from Github, it does not have node modules in it .So, you can do :
npm install
It will look into the package.json file and install all the necessary dependencies.
Components
Components are the building blocks of any react application. It is the heart of react application. A react application is made up of several component heart of react application. A navbar,sidebar or an article might be a component. It is our job as a react developer to make all of these components and then render it to the DOM and show it in the webpage by react.
Components contain:
All of their template and all of their logic. For example, a navbar component will contain all the html which makes that component but also any javascript logic like a function which maybe runs when we click on the logout button. React components allow these things to be done really easily.
Now, Let’s discuss about root component. A root component is a component that gets rendered to the DOM .In this case i.e in our starter project the root component is App.js.
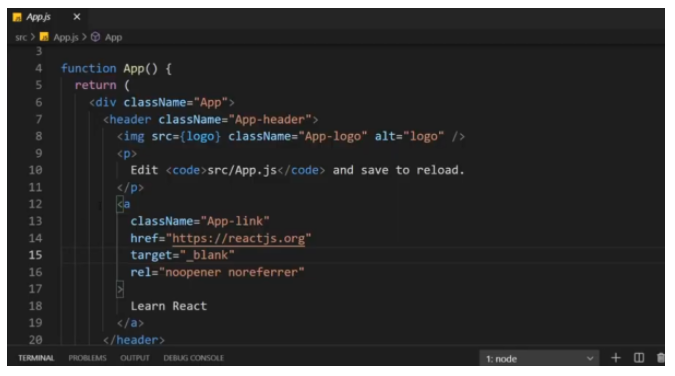
In case of react, it is JSX that helps us to create html templates and logic together. So, all of the code that you see here is written in JSX. In the background, a transpiler called babel converts all of these JSX into html templates when we save the file and then renders it to DOM.
One of the key difference between html and JSX is, that in our html code we used to write class but here in the jsx we are using camel case standard i.e for the classes we are using className.It is because class is a reserved keyword in javascript and at the end of day we have to include javascript as well and in the end when it is finally converted into html , if you inspect you will see it is written as class.
Quick note: If you are using react version less than 17, you will need to import react in your file.
At the end of our code , we always export our file. It is because it makes easy for us to use that component inside some other files.
A component in nutshell is a function and we always return something inside that function and generally that’s going to be JSX template and in the end we export that component so that we can use it somewhere else.
Dynamic Values in Templates
(Using JSX)
We can also have dynamic values in our templates.
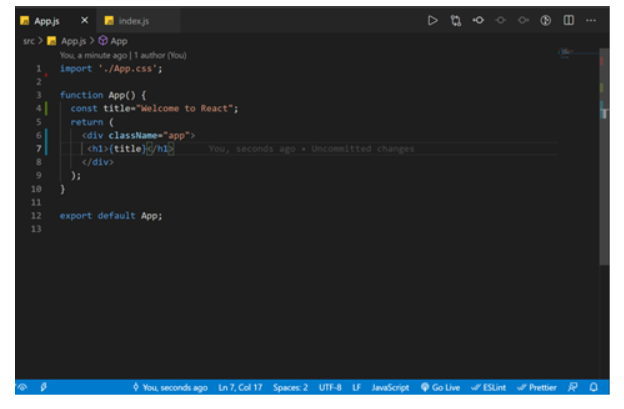
We can define a variable inside the function and inside the curly braces we use that variable. JSX allows us to use dynamic values within curly braces.
We can always define any valid javascript function inside our component and use it in our template.
We can return multiple variables for that matter.
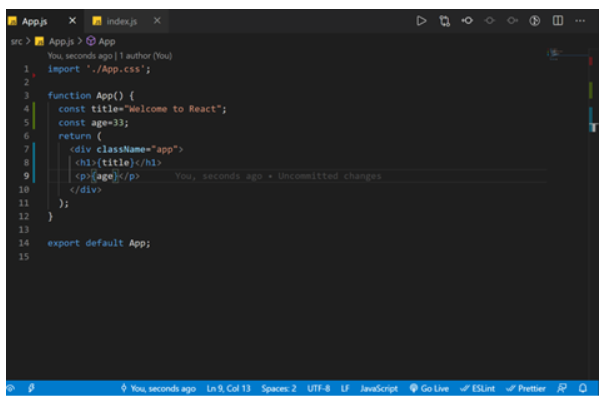
In this case, it is a number and in the previous case it was a string , but it does not matter whether we pass a string or a number it will eventually get converted to string .
The only thing that we cannot pass to these curly braces are objects and Booleans.
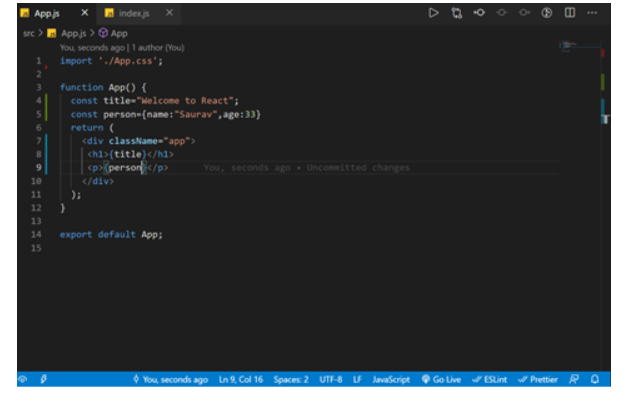
It will throw error on us.
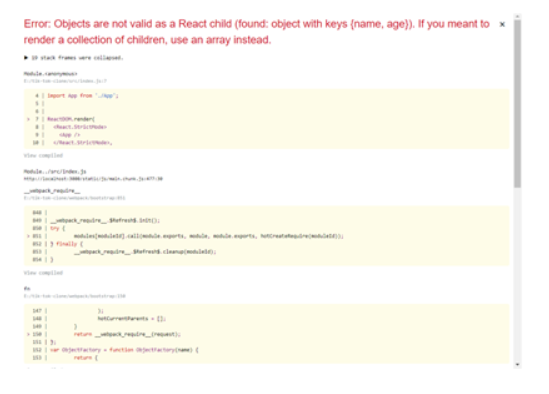
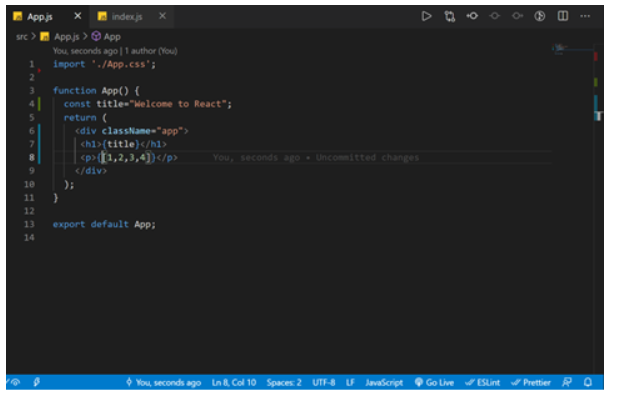
We can also pass arrays in the curly braces directly.
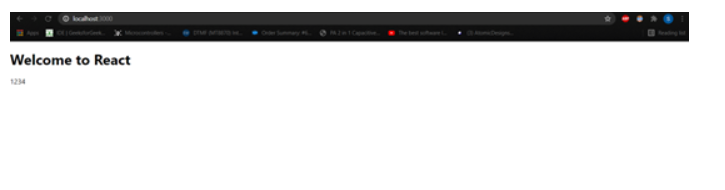
The elements in the array gets converted to string.
We can also use these dynamic values inside html attributes .
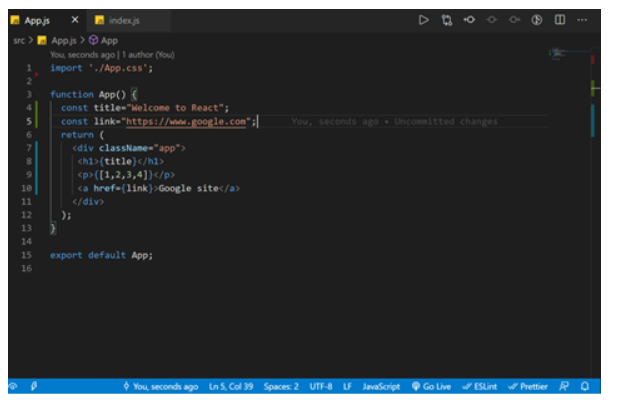
This how we can use dynamic values and it is very useful. You will realise it as you will progress through the course.
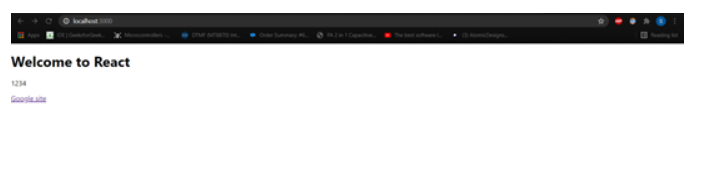
Understanding Lists in React
We are now moving on to make some serious templates for our blog app.
Ideally , we need to have list of blogs which we can output in the template .
We need to use some state to do that . In this case, we are going to use useState Hook .
It is required as the data might change in some time , we might delete a blog , update the blog , in that case , react should be able to update the DOM when that happens.
At the top in our function , we will create some states , we will destructure it into an array and return array of objects
const Home = () => {
const [blogs,setBlogs]= useState([
{title: 'My Blog Website',body:'lorem ipsum...',author:'Sayuri',id:1},
{title: 'Frontend Developers',body:'lorem ipsum...',author:'Saurav',id:2},
{title: 'How to become a Frontend Developer?',body:'lorem ipsum...',author:'Devyani',id:3}
]
);
No Comments