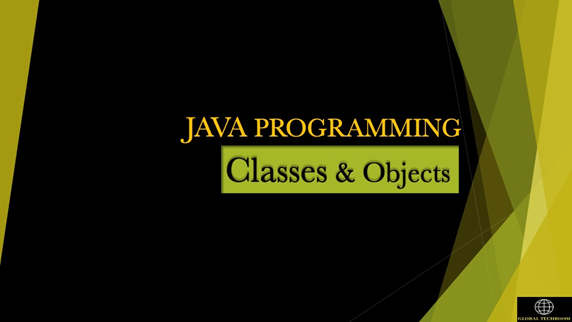
In this article, we will get familiar with concepts of classes and objects with demo of real-world examples.
Please read the entire article, carefully so that you will be clear with core concepts.

For an object-oriented language like java, an application program is developed using classes and objects.
The structure of a java program is made up of classes. Let’s move on to details of Java classes.
Classes in Java:
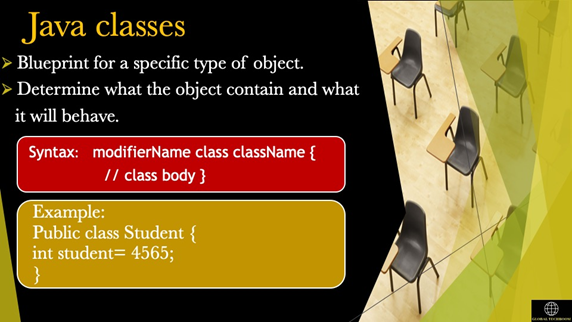
A Class is a blueprint of objects created.
It is like a building a prototype of a house, which is planned to build in future. It will give an idea about the final product. The prototype would tell you what the kitchen should like, or the living room will be.
To create a class in java, you should use keyword ‘class’.
Components of Class in Java:

The next few topics covers the main components of class which are required to write any java program.
The functionality of the members of a class can be protected from other parts of the program by the presence of modifiers.
Modifiers limits the visibility of the classes, fields, constructors or methods in the Java program.
By convention, a class name should begin with a capital letter and following characters should be lowercase.
The variables of a class that stores a data or value are known as fields.
A constructor can be used to create an object.
If you don’t explicitly define a constructor, the compiler automatically adds a default constructor inside the class.
A method can be used to define an action or behavior of the class.
The main method provides an entry point to start execution of any program.
Modifiers – Access Modifiers:

Modifiers in Javalimits the visibility of the classes, fields, constructors or methods in the Java program.
Functionality of the members of a class or a class itself can be protected from other parts of the program by the presence or absence of modifiers.
These modifiers can be divided into two categories: access modifiers and non-access modifiers.
The members of class can be public, private, default, and protected. All these are the access modifiers.
The access modifiers are also known as visibility modifiers. Java provides four access modifiers in object-oriented languages. They are private, default, protected, and public.
Private members (field, method or constructor) of a class cannot be accessed from outside the class. They are accessible only within the class.
A class cannot be private except for inner classes.
If we declare a method as private, it behaves as a method declared as final. We cannot call the private method from outside the class.
Modifiers – Non-Access Modifiers:
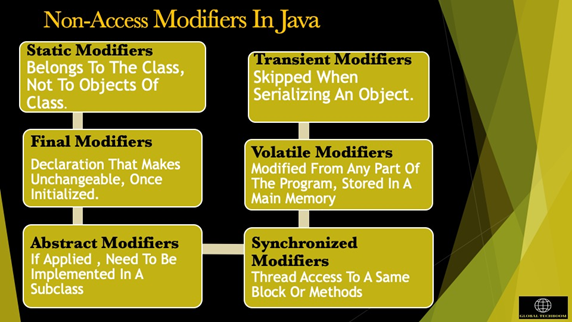
We shall see the classification of Non-Access Modifiers in Java
1. Static modifier: -Static modifier makes class member independent of any object of that class.
Variables and methods declared static can be accessed via the class name
1. A static block gets executed only once when the class is first instantiated.
2. Final modifier: -Final modifier to a variable declaration makes that variable unchangeable
Final modifier for methods is used to prevent a method from being overridden
3. Abstract modifier: - Abstract modifier is used to define methods that will be implemented in a subclass. It used to suggest that some functionality should be implemented in a subclass
4. Transient Modifier: - When a variable is declared as transient, that means that its value isn't saved when the object is stored in memory.
5.Let’s see Volatile Modifier: - As volatile variable is stored in main memory, it can be changed unexpectedly by some other part of the program
6.Last one, Synchronized Modifier: - Synchronized keyword is used when more threads need to use the same resource.
Fields / Variables:

Variable holds the value during the execution of Java program. Each variable in Java has a specific type.
all the variables must be declared to the compiler before they can be used in the program.
There are three types of variables in java:
- Local variables.
- Instance variables.
- Class or Static variables.
A variable which is declared and used inside the body of constructors, methods or blocks known as local variables. Local variables must be assigned a value at the time of creating.
The variables which are declared inside the class but outside the body of the method, constructor or any block are called instance variables. They are available for the entire class methods, constructors, and blocks.
The variable which is declared with static keyword is known as static variable. A static variable is called class variable, because it is associated with the class.
Constructor in Java:
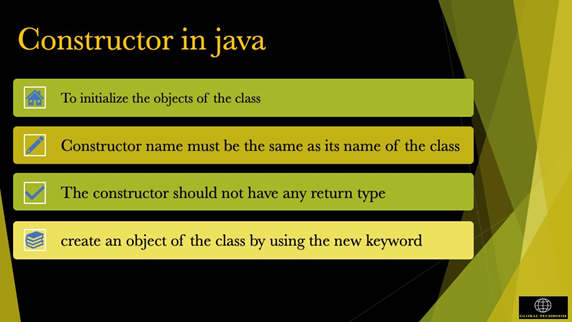
The purpose of the constructor is to initialize the objects of the class.
It is invoked when an instance of a class is created using the new operator.
Constructor name must be the same as its name of the class.
Whenever you create an object/instance of a class, the constructor will be automatically called by the JVM. If you don’t define any constructor inside the class, the compiler automatically creates a default constructor at compile-time.
The constructor should not have any return type.
When we create an object of the class by using the new keyword, a constructor is automatically called.
Program example for constructor:

Let see an example: In this program, Public Employee constructor is created with employee id & employee name as local variables
We can create Constructor in different ways as mentioned here and the first one is the most common one!!
Methods in Java:
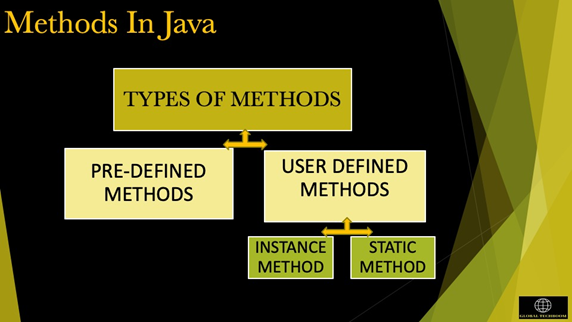
Method is a set of code used to write the logic of the applications like simple example of addition of two numbers.
The methods must be declared inside the class.
When a method is called, it returns the value.
In general, methods have six fundamental parts such as
- Modifiers
- Method name
- Return type
- Parameter list
- Exception list
- Body of program
The method modifier defines the access type of a method.
If the method is abstract, the implementation is omitted, and the method body is replaced with a single semicolon.
The return type defines the type of data that comes back from the method after calling it.
Predefined methods are those methods that are already defined in the Java API (Application Programming Interface) to use in an application.
User-defined methods are those methods which are defined inside a class to perform special task or function in an application.
instance methods represent behaviors of the objects. Therefore, they are linked with an object.
Static method is also known as a class method. It is used to implement the behavior of the class itself. Static methods load into the memory during class loading and before object creation. To call a static method, we use dot operator with the class name.
Objects in Java:

Object is a software module that has state and behavior. An object's state is contained in its member variables and its behavior is implemented through its methods. Generally, Java programs that you write will create many objects from written codes known as classes.
Because software objects represent after real-world objects, you can represent these in programs using software objects. The characteristics of java objects are state, behavior and identity
Program example for object creation:

In this example we have two separate classes written and define main() method in another class. By creating Dog object in main class, it is assigning values.
Java Class is an entity that determines how Java Objects will behave and what objects will contain
A Java object is a self-contained component which consists of methods and properties to make certain type of data useful.
Demo Program:
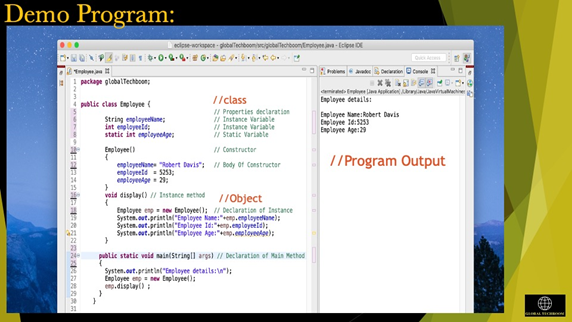
Let’s move on to a demo program
The starting point for a java program is the main method in the class.
Out of the elements declared inside the class body, the static variable, static block, and static method are executed first during loading of class file.
The “public” is the access modifier used in this program. process of creating an object of a particular class (Employee) is called instantiating of an object.
Once a class has been created, any number of objects belonging to that class can be created.
No Comments