Android AsyncTask going to do background operation on background thread and update on main thread. In android we cant directly touch background thread to main thread in android development. asynctask help us to make communication between background thread to main thread.
Methods of AsyncTask
- onPreExecute() − Before doing background operation we should show something on screen like progressbar or any animation to user. we can directly comminicate background operation using on doInBackground() but for the best practice, we should call all asyncTask methods .
- doInBackground(Params) − In this method we have to do background operation on background thread. Operations in this method should not touch on any mainthread activities or fragments.
- onProgressUpdate(Progress…) − While doing background operation, if you want to update some information on UI, we can use this method.
- onPostExecute(Result) − In this method we can update ui of background operation result.
The process to fetch data from API
1.Open the activity_main.xml file and add the components as shown below.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="30dp"
tools:context=".MainActivity">
<Button
android:id="@+id/displayData"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="20dp"
android:text="Click to Show Users"
android:textColor="@color/cardview_dark_background"
android:textSize="18sp" />
<TextView
android:id="@+id/results"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="50dp"
android:visibility="gone"
android:textColor="@color/design_default_color_primary"
android:textSize="18sp" />
</LinearLayout>
2.Define the URL and the UI components at the top of the Main_activity.java file.
public class MainActivity extends AppCompatActivity {
String myUrl = "https://api.mocki.io/v1/a44b26bb";
TextView resultsTextView;
ProgressDialog progressDialog;
Button displayData;
//the rest of the code
}
Next, use findViewById() to hook the components on the onCreate method.
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
resultsTextView = (TextView) findViewById(R.id.results);
displayData = (Button) findViewById(R.id.displayData);
}
Create the AsyncTask method for downloading network data from the API, and implement the onPreExecute(), doInBackground(), and onPostExecute() methods.
public class MyAsyncTasks extends AsyncTask<String, String, String> {
@Override
protected void onPreExecute() {
super.onPreExecute();
// display a progress dialog to show the user what is happening
@Override
protected String doInBackground(String... params) {
// Fetch data from the API in the background.
}
@Override
protected void onPostExecute(String s) {
// show results
}
}
On the onPreExecute() method, display a progress dialog that lets the user know what is happening.
@Override
protected void onPreExecute() {
super.onPreExecute();
// display a progress dialog for good user experiance
progressDialog = new ProgressDialog(MainActivity.this);
progressDialog.setMessage("processing results");
progressDialog.setCancelable(false);
progressDialog.show();
}
On the doInBackground method, create a URL connection and read the data from the API. Surround the operation with try-catch clauses to catch any exceptions that might occur when fetching data from the API.
@Override
protected String doInBackground(String... params) {
// Fetch data from the API in the background.
String result = "";
try {
URL url;
HttpURLConnection urlConnection = null;
try {
url = new URL(myUrl);
//open a URL coonnection
urlConnection = (HttpURLConnection) url.openConnection();
InputStream in = urlConnection.getInputStream();
InputStreamReader isw = new InputStreamReader(in);
int data = isw.read();
while (data != -1) {
result += (char) data;
data = isw.read();
}
// return the data to onPostExecute method
return result;
} catch (Exception e) {
e.printStackTrace();
} finally {
if (urlConnection != null) {
urlConnection.disconnect();
}
}
} catch (Exception e) {
e.printStackTrace();
return "Exception: " + e.getMessage();
}
return result;
}
On the onPostExecute() method, dismiss the progress dialog and display the results to the user with a text view.
@Override
protected void onPostExecute(String s) {
// dismiss the progress dialog after receiving data from API
progressDialog.dismiss();
try {
JSONObject jsonObject = new JSONObject(s);
JSONArray jsonArray1 = jsonObject.getJSONArray("users");
JSONObject jsonObject1 =jsonArray1.getJSONObject(index_no);
String id = jsonObject1.getString("id");
String name = jsonObject1.getString("name");
String my_users = "User ID: "+id+"\n"+"Name: "+name;
//Show the Textview after fetching data
resultsTextView.setVisibility(View.VISIBLE);
//Display data with the Textview
resultsTextView.setText(my_users);
} catch (JSONException e) {
e.printStackTrace();
}
}
Finally, set an onClickLIstener on the button and execute MyAsyncTasks when a user clicks the button.
displayData.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// create object of MyAsyncTasks class and execute it
MyAsyncTasks myAsyncTasks = new MyAsyncTasks();
myAsyncTasks.execute();
}
});
Preview of app
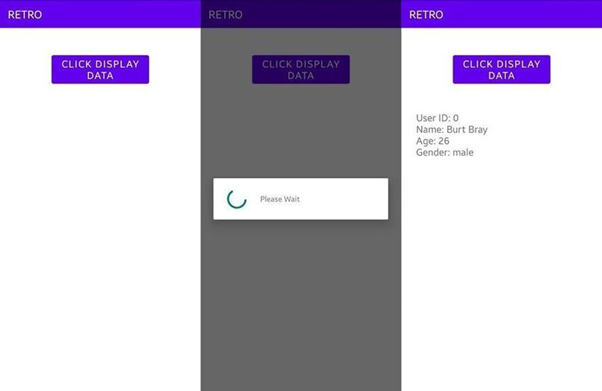
No Comments