XML represents Extensible Mark-up Language.XML is a mainstream design and usually utilized for sharing information on the web. This section discloses how to parse the XML document and concentrate important data from it.
Android gives three sorts of XML parsers which are DOM,SAX and XMLPullParser. Among every one of them android suggest XMLPullParser in light of the fact that it is productive and simple to utilize. So we will utilize XMLPullParser for parsing XML.
The initial step is to distinguish the fields in the XML information where you are keen on. For instance. In the XML given underneath we keen on getting temperature as it were.
<?xml version="1.0"?>
<current>
<city id="2643743" name="London">
<coord lon="-0.12574" lat="51.50853"/>
<country>GB</country>
<sun rise="2013-10-08T06:13:56" set="2013-10-08T17:21:45"/>
</city>
<temperature value="289.54" min="289.15" max="290.15" unit="kelvin"/>
<humidity value="77" unit="%"/>
<pressure value="1025" unit="hPa"/>
</current>
XML – Elements
An xml file consists of many components. Here is the table defining the components of an XML file and their description
Component & description |
---|
Prolog An XML file starts with a prolog. The first line that contains the information about a file is prolog |
Events An XML file has many events. Event could be like this. Document starts , Document ends, Tag start , Tag end and Text e.t.c |
Text Apart from tags and events, and xml file also contains simple text. Such as GB is a text in the country tag. |
Attributes Attributes are the additional properties of a tag such as value e.t.c |
A part from the these strategies, there are different techniques given by this class to better parsing XML records. These techniques are recorded below.
getAttributeCount() This method just Returns the number of attributes of the current start tag |
getAttributeName(int index) This method returns the name of the attribute specified by the index value |
getColumnNumber() This method returns the Returns the current column number, starting from 0. |
getDepth() This method returns Returns the current depth of the element. |
getLineNumber() Returns the current line number, starting from 1. |
getNamespace() This method returns the name space URI of the current element. |
getPrefix() This method returns the prefix of the current element |
getName() This method returns the name of the tag |
getText() This method returns the text for that particular element |
isWhitespace() This method checks whether the current TEXT event contains only whitespace characters. |
Following is the content of the modified main activity file MainActivity.java
import java.io.InputStream;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
public class MainActivity extends Activity {
TextView tv1;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tv1=(TextView)findViewById(R.id.textView1);
try {
InputStream is = getAssets().open("file.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(is);
Element element=doc.getDocumentElement();
element.normalize();
NodeList nList = doc.getElementsByTagName("employee");
for (int i=0; i<nList.getLength(); i++) {
Node node = nList.item(i);
if (node.getNodeType() == Node.ELEMENT_NODE) {
Element element2 = (Element) node;
tv1.setText(tv1.getText()+"\nFirst Name: " + getValue("fname", element2)+"\n");
tv1.setText(tv1.getText()+"Last Name: " + getValue("lname", element2)+"\n");
tv1.setText(tv1.getText()+"-----------------------");
}
}
} catch (Exception e) {e.printStackTrace();}
}
private static String getValue(String tag, Element element) {
NodeList nodeList = element.getElementsByTagName(tag).item(0).getChildNodes();
Node node = nodeList.item(0);
return node.getNodeValue();
}
}
Following is the content of Assets/file.xml
<?xml version="1.0"?>
<records>
<employee>
<fname>John</fname >
<lname>Doe</lname >
<salary>$500</salary>
</employee>
<employee>
<fname >Max</fname >
<lname >Warden</lname >
<salary>$350</salary>
</employee>
</records>
Following is the modified content of the xml res/layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</RelativeLayout>
Following is the content of AndroidManifest.xml file
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.new.appxapps.myapplication" >
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Preview on application
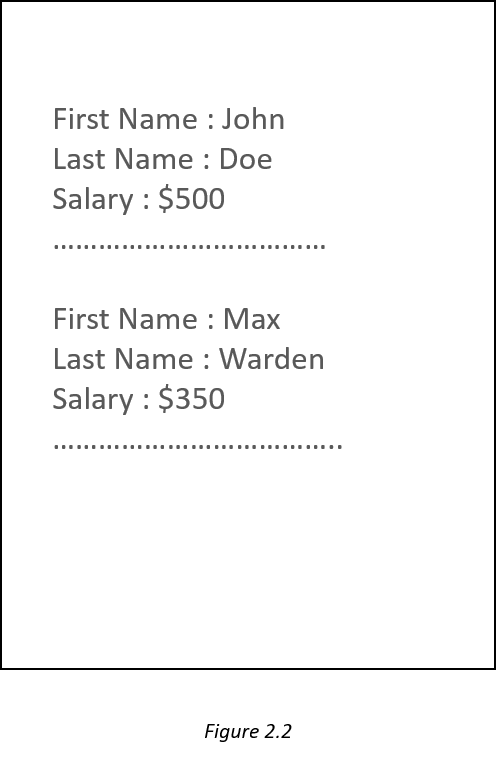
No Comments