Preferences are fundamentally used to monitor the application alongside the client preferences. An inclination is a straightforward key-esteem pair with a particular information type for the worth. The undertaking of inclination key is to extraordinarily distinguish an inclination and then again values store the inclination esteems.
For preferences, The data types that can be chosen are as follows:
- Boolean Types
- Integer Types
- Float Types
- String Types
- Long Types
There are three APIs from which one can be chosen to get access to the preferences. These three APIs are:
- getPreference(): It is used within the Activity, to access activity-specific preference.
- getSharedPreference(): It is used from the Activity or some other application context. Its use is to access application-level preferences.
- getDefaultSharedPreference(): It is used on the PreferenceManager, to get the shared preferences that goes with the overall Android preference framework.
To use it, we need to call the method getSharedPreferences() that returns a SharedPreference instance pointing to the file containing the values of preferences. This is done as follows:
SharedPreferences sharedpreference_name = getSharedPreferences(String name, MODE_PRIVATE);
Android Shared Preferences methods
- contains( String key): It checks if the preference contains a preference or not.
- edit(): It creates a new Editor for preferences, to enable modifications to the data and commit changes back to the SharedPreferences atomically.
- getAll(): It retrieves all the values from the preferences.
- getBoolean(String key, boolean defValue): It retrieves a boolean value from the preferences.
- getFloat(String key, float defValue): It retrieves a float value from the preferences.
- getInt(String key, int defValue): It retrieves an int value from the preferences.
- getLong(String key, long defValue): It retrieves a long value from the preferences.
- getString(String key, String defValue): It retrieves a String value from the preferences.
- getStringSet(String key, Set defValue): It retrieves a Set of String values from the preferences.
Shared preference in Android Step by Step Implementation
1. First, we’ll create a new project and name it.
2. Now write the following code in the activity_main.xml file.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginLeft="90dp"
android:layout_marginTop="30dp"
android:text="DataFlair "
android:textColor="#00574B"
android:textSize="50dp"
android:textStyle="bold" />
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:layout_marginTop="80dp"
android:text="Enter your UserName" />
<EditText
android:id="@+id/Name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:ems="10" />
<TextView
android:id="@+id/text2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:text="Enter your Password" />
<EditText
android:id="@+id/password"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:ems="10"
android:inputType="textPassword" />
<Button
android:id="@+id/Login"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:text="Login" />
</LinearLayout>
3. Now, write the following code in the MainActivity.java file.
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
EditText username, pass;
Button Login_Button;
SharedPreferences Shared_pref;
Intent intent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
username = findViewById(R.id.Name);
pass = findViewById(R.id.password);
Login_Button = findViewById(R.id.Login);
Shared_pref = getSharedPreferences("details", MODE_PRIVATE);
intent = new Intent(MainActivity.this, secondActivity.class);
if (Shared_pref.contains("username") && Shared_pref.contains("password")) {
startActivity(intent);
}
Login_Button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = MainActivity.this.username.getText().toString();
String password = pass.getText().toString();
if (username.equals("joy") && password.equals("123456")) {
SharedPreferences.Editor editor = Shared_pref.edit();
editor.putString("username", username);
editor.putString("password", password);
editor.commit();
Toast.makeText(getApplicationContext(), "Logged in", Toast.LENGTH_SHORT).show();
startActivity(intent);
} else {
Toast.makeText(getApplicationContext(), "Enter Right Credentials", Toast.LENGTH_SHORT).show();
}
}
});
}
}
4. Now create a new layout file second.xml in the resource/ layout/. And write the following code there
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/res_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="170dp"
android:textSize="22dp" />
<Button
android:id="@+id/LogOut"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="25dp"
android:text="Log Out" />
</LinearLayout>
5. Create a new java file secondActivity.java in the same folder as that of MainActivity.java. Write the following code in the secondActivity.java file
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class secondActivity extends AppCompatActivity {
SharedPreferences newPreference;
Intent newIntent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.second);
TextView result = findViewById(R.id.res_text);
Button LogOut_btn = findViewById(R.id.LogOut);
newPreference = getSharedPreferences("user_details", MODE_PRIVATE);
newIntent = new Intent(secondActivity.this, MainActivity.class);
result.setText("Welcome, " + newPreference.getString("username", null));
LogOut_btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
SharedPreferences.Editor edit = newPreference.edit();
edit.clear();
edit.commit();
startActivity(newIntent);
}
});
}
}
6. Write the following in the Manifest.xml File
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.dataflair.mysharedpreference">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity
android:name=".secondActivity"
android:label="Shared Preferences - Details"></activity>
</application>
</manifest>
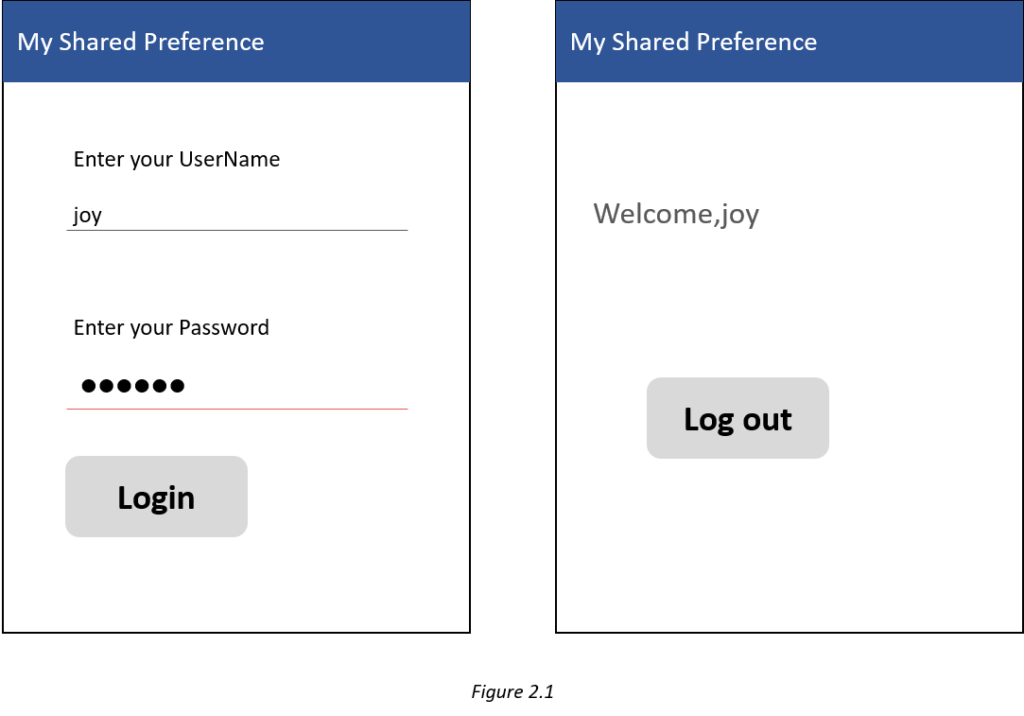
No Comments